Why does in the DetailsActivity are values not passed from the MainActivity in AndroidStudio?
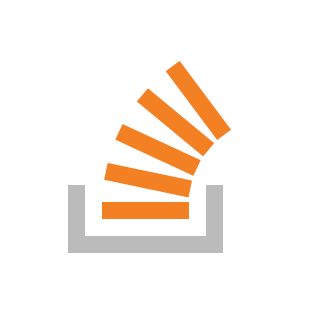
The problem is: Destination, date and notes are not displayed in the DetailsActivity, only the trip type is displayed. I think there is a problem in the Intent but honestly I can't figure out which one and after several days of intensive study I kindly ask you for help. Thank you in advance.
PS. I'll try to post the code. If necessay I'll post activity.xml and so on.
//MainActivity
package com.example.mytravelplanner;
import android.app.DatePickerDialog;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Spinner;
import android.widget.TextView;
import android.widget.Toast;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Locale;
public class MainActivity extends AppCompatActivity {
private EditText editTextDestination;
private Spinner spinnerTravelType;
private Button buttonSelectDate;
private TextView textViewSelectedDate;
private EditText editTextNote;
private Button buttonAddDestination;
private RecyclerView recyclerView;
private DestinationsAdapter destinationsAdapter;
private ArrayList<Destination> destinationsList;
private String selectedDate = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
// Collega le view dal layout (activity_main.xml)
editTextDestination = findViewById(R.id.editTextDestination);
spinnerTravelType = findViewById(R.id.spinnerTravelType);
buttonSelectDate = findViewById(R.id.buttonSelectDate);
editTextNote = findViewById(R.id.editTextNote);
buttonAddDestination = findViewById(R.id.buttonAddDestination);
recyclerView = findViewById(R.id.recyclerViewDestinations);
textViewSelectedDate = findViewById(R.id.textViewSelectedDate);
// Inizializza la lista delle destinazioni, della RecyclerView e l'adapter
destinationsList = new ArrayList<>();
destinationsAdapter = new DestinationsAdapter(destinationsList); // gestisce il contenuto della lista
recyclerView.setLayoutManager(new LinearLayoutManager(this)); // imposta il layout manager per visualizzare le destinazioni in una lista verticale
recyclerView.setAdapter(destinationsAdapter);
//Aggiungi un listener di click sugli elementi della lista
// Configurazione dello spinner
ArrayAdapter<CharSequence> spinnerAdapter = ArrayAdapter.createFromResource(
this,
R.array.travel_types, // Array definito in strings.xml
android.R.layout.simple_spinner_item // Layout predefinito per gli elementi del spinner
);
spinnerAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
spinnerTravelType.setAdapter(spinnerAdapter);
// Gestione del pulsante "Seleziona Data" attraverso un OnClickListener
buttonSelectDate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showDatePicker();
}
});
// Gestione del pulsante "Aggiungi Destinazione" attraverso un OnClickListener
buttonAddDestination.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String travelType = spinnerTravelType.getSelectedItem().toString();
// Verifica che i campi obbligatori siano compilati
if (editTextDestination.getText().toString().isEmpty() ||
selectedDate.isEmpty() ||
travelType.isEmpty()) {
Toast.makeText(MainActivity.this, "Compila tutti i campi obbligatori", Toast.LENGTH_SHORT).show();
return;
}
// Log per debug
Log.d("MainActivity", "Aggiunta destinazione: " + editTextDestination.getText().toString());
// Aggiungi la destinazione alla lista
addDestination();
// Crea un Intent per passare alla DetailsActivity
Intent intent = new Intent(MainActivity.this, DetailsActivity.class);
intent.putExtra("destinationName", destinationsList.toString());
intent.putExtra("travelType", travelType);
intent.putExtra("travelDate", selectedDate);
intent.putExtra("note", editTextNote.getText().toString());
startActivity(intent);
}
});
}
// Metodo per selezionare la data
private void showDatePicker() {
Log.d("DatePicker", "showDatePicker chiamato");
Calendar calendar = Calendar.getInstance();
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH);
int day = calendar.get(Calendar.DAY_OF_MONTH);
DatePickerDialog datePickerDialog = new DatePickerDialog(MainActivity.this,
(view, year1, month1, dayOfMonth) -> {
Calendar selectedCalendar = Calendar.getInstance();
selectedCalendar.set(year1, month1, dayOfMonth);
// Formatta la data
SimpleDateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy", Locale.getDefault());
selectedDate = dateFormat.format(selectedCalendar.getTime());
// Aggiorna la TextView con la data selezionata
textViewSelectedDate.setText("Data Selezionata: " + selectedDate);
},
year, month, day);
datePickerDialog.show();
}
// Metodo per aggiungere una destinazione alla lista
private void addDestination() {
String destinationName = editTextDestination.getText().toString();
String travelType = spinnerTravelType.getSelectedItem().toString();
String date = selectedDate;
String note = editTextNote.getText().toString();
// Log per vedere se destinationName è correttamente popolato
Log.d("MainActivity", "Destination Name: " + destinationName); // Qui aggiungi il log
// Verifica che i campi obbligatori siano compilati
if (destinationName.isEmpty() || travelType.isEmpty() || date.isEmpty()) {
Toast.makeText(this, "Compila tutti i campi", Toast.LENGTH_SHORT).show();
return;
}
// Crea un nuovo oggetto Destination
Destination newDestination = new Destination(destinationName, travelType, date, note);
destinationsList.add(newDestination); // Aggiungi l'oggetto Destination alla lista
destinationsAdapter.notifyItemInserted(destinationsList.size() - 1); // Notifica l'adapter che ci sono nuovi dati per aggiornare la RecyclerView
// Pulisce i campi
editTextDestination.setText("");
spinnerTravelType.setSelection(0);
selectedDate = "";
textViewSelectedDate.setText("Nessuna Data Selezionata");
editTextNote.setText("");
// Mostra un messaggio di conferma
Toast.makeText(this, "Destinazione Aggiunta!", Toast.LENGTH_SHORT).show();
}
}
//DetailsActivity
package com.example.mytravelplanner;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
public class DetailsActivity extends AppCompatActivity {
private TextView textViewDestination;
private TextView textViewTravelType;
private TextView textViewDate;
private TextView textViewNote;
private Button buttonBack;
private Button buttonShare;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_details);
// Collega le view dal layout (activity_details.xml)
textViewDestination = findViewById(R.id.textViewDestinationName);
textViewTravelType = findViewById(R.id.textViewType);
textViewDate = findViewById(R.id.textViewTravelDate);
textViewNote = findViewById(R.id.textViewTravelNote);
// Collega il pulsante di ritorno alla MainActivity
buttonBack = findViewById(R.id.buttonBack);
// Collega il pulsante di condivisione della destinazione
buttonShare = findViewById(R.id.buttonShare);
// Listener per il pulsante di condivisione
buttonShare.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Ottieni i dati da condividere della destinazione dalla MainActivity
String destinationName = textViewDestination.getText().toString();
String travelType = textViewTravelType.getText().toString();
String date = textViewDate.getText().toString();
String note = textViewNote.getText().toString();
// Crea una stringa che contiene i dati da condividere
String message = "Destinazione: " + destinationName + "\n" +
"Tipo di viaggio: " + travelType + "\n" +
"Data: " + date + "\n" +
"Note: " + note;
// Usa un Intent implicito per la condivisione
Intent shareIntent = new Intent(Intent.ACTION_SEND);
shareIntent.setType("text/plain");
shareIntent.putExtra(Intent.EXTRA_TEXT, message);
// Avvia il chooser per selezionare l'applicazione di condivisione
startActivity(Intent.createChooser(shareIntent, "Condividi Destinazione"));
}
});
// Recupera i dati passati dalla MainActivity
Bundle extras = getIntent().getExtras();
if (extras != null) {
String destinationName = extras.getString("destinationName");
String travelType = extras.getString("travelType");
String travelDate = extras.getString("travelDate");
String note = extras.getString("note");
//Log per verificare i dati ricevuti
Log.d("DetailsActivity", "Received Destination Name: " + destinationName);
// Controlla se i dati non sono nulli o vuoti e imposta il testo
if (destinationName != null && !destinationName.isEmpty()) {
textViewDestination.setText("Destinazione: " + destinationName);
} else {
textViewDestination.setText("Destinazione non disponibile");
}
if (travelType != null && !travelType.isEmpty()) {
textViewTravelType.setText("Tipo di viaggio: " + travelType);
} else {
textViewTravelType.setText("Tipo di viaggio non disponibile");
}
if (travelDate != null && !travelDate.isEmpty()) {
textViewDate.setText("Data: " + travelDate);
} else {
textViewDate.setText("Data non disponibile");
}
if (note != null && !note.isEmpty()) {
textViewNote.setText("Note: " + note);
} else {
textViewNote.setText("Note non disponibili");
}
}
// Listener per il pulsante di ritorno alla MainActivity
buttonBack.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish(); // Termina l'Activity corrente
}
});
// Log per il debug (spostato dopo l'inizializzazione delle viste)
Log.d("DetailsActivity", "TextView Destination initialized: " + textViewDestination);
}
}
//DestinationsAdapter
package com.example.mytravelplanner;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
public class DestinationsAdapter extends RecyclerView.Adapter<DestinationsAdapter.DestinationViewHolder>{
private final ArrayList<Destination> destinationsList; //Lista delle destinazioni da visualizzare
//Costruttore per inizializzare la lista delle destinazioni
public DestinationsAdapter(ArrayList<Destination> destinationsList){
this.destinationsList = destinationsList;
}
//Classe interna per il ViewHolder
public static class DestinationViewHolder extends RecyclerView.ViewHolder{
public TextView textViewDestination;
public TextView textViewTravelType;
public TextView textViewDate;
public TextView textViewNote;
public DestinationViewHolder(View itemView){
super(itemView);
//Collega le view dal layout (destination_item.xml)
textViewDestination = itemView.findViewById(R.id.textViewDestination);
textViewTravelType = itemView.findViewById(R.id.textViewTravelType);
textViewDate = itemView.findViewById(R.id.textViewDate);
textViewNote = itemView.findViewById(R.id.textViewNote);
}
}
public DestinationViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
//Infla il layout del singolo elemento della lista
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.destination_item, parent, false);
return new DestinationViewHolder(view);
}
//Metodo onBindViewHolder per impostare i dati in ogni elemento della lista
@Override
public void onBindViewHolder(@NonNull DestinationViewHolder holder, int position) {
// Ottieni l'oggetto Destination alla posizione corrente
Destination destination = destinationsList.get(position);
//Imposta i dati nel ViewHolder
holder.textViewDestination.setText(destination.getName());
holder.textViewTravelType.setText(destination.getTravelType());
holder.textViewDate.setText(destination.getDate());
holder.textViewNote.setText(destination.getNote());
}
public int getItemCount() {
/*Restituisce il numero totale di elementi nella lista, utilizzato da RecyclerView
per sapere quanti elementi mostrare
*/
return destinationsList.size();
}
}
//Destination
package com.example.mytravelplanner;
public class Destination {
private String name;
private String travelType;//tipo di viaggio (es.Lavoro, studio ecc.)
private String date;
private String note;
//Costruttore
public Destination (String name, String travelType, String date, String note) {
this.name = name;
this.travelType = travelType;
this.date = date;
this.note = note;
}
//Metodi GETTER
public String getName(){
return name;
}
public String getTravelType(){
return travelType;
}
public String getDate(){
return date;
}
public String getNote(){
return note;
}
//Metodi SETTER
public void setName(String name){
this.name = name;
}
public void setTravelType(String travelType){
this.travelType = travelType;
}
public void setDate(String date) {
this.date = date;
}
public void setNote(String note){
this.note = note;
}
//Metodo toString
public String toString(){
return "Destination{" +
"name='" + name + '\'' +
", travelType='" + travelType + '\'' +
", date='" + date + '\'' +
", note='" + note + '\'' +
'}';
}
}
I'm expecting to solve this little problem
Source: View source