How to resolve Google Play rejection for "Prominent Disclosure & Consent Requirement" in a React Native app?
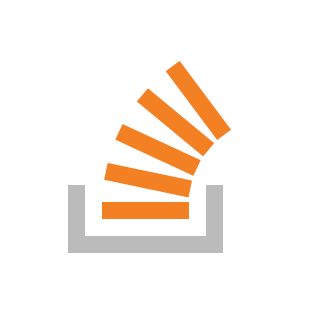
I recently updated my React Native app, but Google Play rejected it for not adhering to the User Data policy, specifically regarding Prominent Disclosure & Consent Requirements. Here’s the rejection message I received:
Your recent app update has been rejected for not adhering to Google Play policies. Address issues and make sure your app meets all policy requirements before resubmitting.
Your app is not compliant with the Prominent Disclosure & Consent Requirement of the User Data policy.
Issue: BACKGROUND_LOCATION permission user consent/runtime permission incorrectly requested before Prominent Disclosure.
I am using the react-native-permissions library to request location permissions in my app. However, it seems like the disclosure is not being displayed prominently enough before requesting the permission.
Here’s a snippet of the code I’m using to request location permissions:
import { request, PERMISSIONS } from 'react-native-permissions';
const requestLocationPermission = async () => {
// Show prominent disclosure here
Alert.alert(
"Location Permission Required",
"This app collects location data to enable background features, even when the app is closed or not in use.",
[
{
text: "OK",
onPress: async () => {
const result = await request(PERMISSIONS.ANDROID.ACCESS_BACKGROUND_LOCATION);
console.log(result);
}
}
]
);
};
What is the correct way to implement a prominent disclosure for location permissions in React Native? Are there any specific UI/UX requirements for prominent disclosure that Google Play expects? How can I test if my app meets the policy requirements before resubmitting it?
Source: View source