Can't display jpg files from the cache of my app Android React-Native
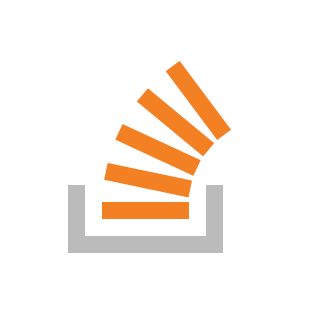
I'm trying to alternate between displaying Reddit and .jpg cache images.
However, when I try to fetch .jpg images from my cache, only one image is recovered and I either can't see the other images or they are totally ignored and I only see Reddit pictures... Please help me solve this I have headaches lol
useEffect(() => {
console.log('Fetching cached images...');
const fetchCachedImages = async () => {
try {
const cacheDir = RNFS.CachesDirectoryPath;
const files = await RNFS.readDir(cacheDir);
const jpegFiles = files.filter(file => file.name.endsWith('.jpg')).map(file => `file://${file.path}`);
setCachedImages(jpegFiles);
console.log('Fetched cached images:', jpegFiles);
} catch (error) {
console.error('Error fetching cached images:', error);
}
};
fetchCachedImages();
}, []);
useEffect(() => {
console.log('Combining Reddit memes and cached images...');
const combined = [];
let redditIndex = 0;
let cachedIndex = 0;
let addReddit = true; // Flag to alternate between Reddit and cached memes
// Limit to first 100 memes
const maxMemes = 100;
// Ensure that we only use 100 memes from both Reddit and cache
while ((redditIndex < memes.length && combined.length < maxMemes) ||
(cachedIndex < cachedImages.length && combined.length < maxMemes)) {
if (addReddit && redditIndex < memes.length && combined.length < maxMemes) {
combined.push({ type: 'reddit', meme: memes[redditIndex] });
console.log(`Added Reddit meme at index ${redditIndex}`);
redditIndex++;
} else if (!addReddit && cachedIndex < cachedImages.length && combined.length < maxMemes) {
combined.push({ type: 'cache', meme: cachedImages[cachedIndex] });
console.log(`Added cached image at index ${cachedIndex}`);
cachedIndex++;
}
addReddit = !addReddit; // Alternate between Reddit and cache
}
setCombinedMemes(combined);
console.log('Combined memes (first 100):', combined);
// Réinitialisation de l'index à 1 lorsque les memes sont combinés
setCurrentIndex(1);
}, [memes, cachedImages]);
useEffect(() => {
console.log('Loading combined memes...');
const loadIndex = async () => {
const storedIndex = await AsyncStorage.getItem('currentIndex');
if (storedIndex) {
setCurrentIndex(parseInt(storedIndex));
} else {
// Si aucun index stocké, initialiser à 1
setCurrentIndex(1);
}
};
loadIndex();
}, []);
I have managed to retrieve the cached images and Reddit memes separately, but I am struggling to properly alternate between them. The app only shows one cached image in the combined array, even though there are multiple cached images in the directory.
When it manages alternating, the cache images are blank...
Source: View source